Testing Typesense search with Testcontainers and .NET
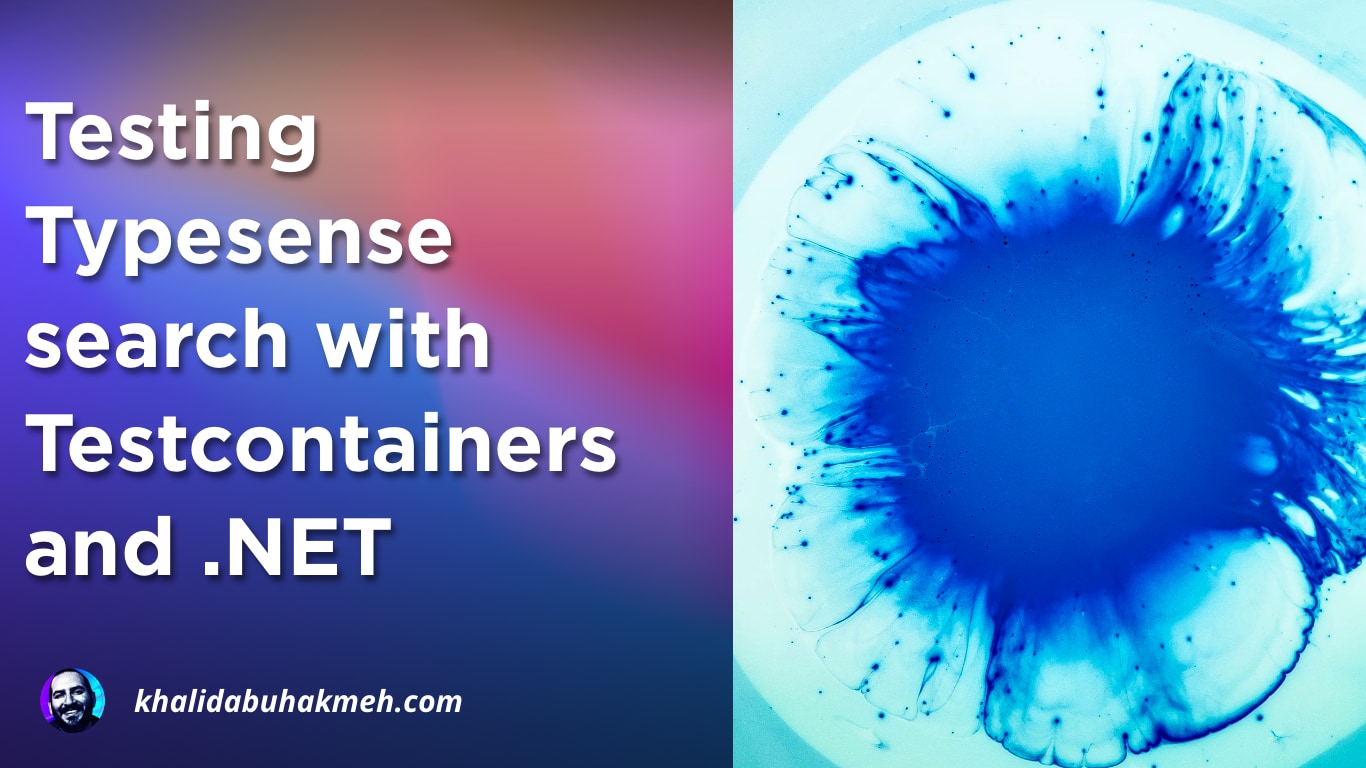
Search is an essential part of any modern application. Without a first-class emphasis on great search, many applicationsaren’t much better than a spreadsheet. Luckily for application developers, we’re spoiled for options for delivering anexcellent search experience to our users. One of the options I’ve been playing with recently is Typesense, which aims tobe an open-source alternative to commercial-service Algolia.In this post, we’ll look at how you can play around with Typesense within the context of .NET using Testcontainers.Testcontainers is a library that makes spinning up containers so simple you’ll wonder how you ever lived without it.Let’s get started.What is TypesenseSearch is challenging to get right, with many commercial options. The most notable commercial offerings includeElasticsearch and Algolia, which come with licensing costs or are search-as-a-service solutions. While great choices intheir own right, the options might not fit your particular goals for finding a search...